图书介绍
INTRODUCTION TO PROGRAMMING WITH C++pdf电子书版本下载
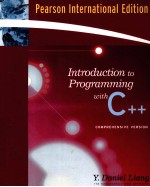
- Y.DANIEL LIANG 著
- 出版社: PEARSON PRENTICE HALL
- ISBN:
- 出版时间:2007
- 标注页数:661页
- 文件大小:156MB
- 文件页数:663页
- 主题词:
PDF下载
下载说明
INTRODUCTION TO PROGRAMMING WITH C++PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如 BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
PART 1 FUNDAMENTALS OF PROGRAMMING 19
Chapter 1 Introduction to Computers,Programs, and C++ 21
1.1 Introduction 22
1.2 What Is a Computer? 22
1.3 Programs 25
1.4 Operating Systems 27
1.5 (Optional) Number Systems 28
1.6 History of C++ 31
1.7 A Simple C++ Program 32
1.8 C++ Program Development Cycle 33
1.9 Developing C++ Programs Using Visual C++ 35
1.10 Developing C++ Programs Using Dev-C++ 41
1.11 Developing C++ Programs from Command Line on Windows 46
1.12 Developing C++ Programs on UNIX 47
Chapter 2 Primitive Data Types and Operations 51
2.1 Introduction 52
2.2 Writing Simple Programs 52
2.3 Reading Input from the Keyboard 54
2.4 Omitting the std: Prefix 55
2.5 Identifiers 56
2.6 Variables 56
2.7 Assignment Statements and Assignment Expressions 57
2.8 Named Constants 59
2.9 Numeric Data Types and Operations 60
2.10 Numeric Type Conversions 66
2.11 Character Data Type and Operations 68
2.12 Case Studies 70
2.13 Programming Style and Documentation 75
2.14 Programming Errors 76
2.15 Debugging 77
Chapter 3 Selection Statements 85
3.1 Introduction 86
3.2 The bool Data Type 86
3.3 i f Statements 87
3.4 Example: Guessing Birth Dates 89
3.5 Logical Operators 91
3.6 if.else Statements 94
3.7 Nested i f Statements 95
3.8 Example: Computing Taxes 97
3.9 Example: A Math Learning Tool 100
3.10 swi tch Statements 101
3.11 Conditional Expressions 104
3.12 Formatting Output 104
3.13 Operator Precedence and Associativity 107
3.14 Enumerated Types 109
Chapter 4 Loops 119
4.1 Introduction 120
4.2 The while Loop 120
4.3 The do-while Loop 124
4.4 The for Loop 126
4.5 Which Loop to Use? 129
4.6 Nested Loops 130
4.7 Case Studies 131
4.8 (Optional) Keywords break and continue 136
4.9 Example: Displaying Prime Numbers 138
4.10 (Optional) Simple File Input and Output 140
Chapter 5 Functions 153
5.1 Introduction 154
5.2 Creating a Function 154
5.3 Calling a Function 155
5.4 void Functions 157
5.5 Passing Parameters by Values 159
5.6 Passing Parameters by References 161
5.7 Overloading Functions 163
5.8 Function Prototypes 165
5.9 Default Arguments 167
5.10 Case Study: Computing Taxes with Functions 168
5.11 Reusing Functions by Different Programs 170
5.12 Case Study: Generating Random Characters 171
5.13 The Scope of Variables 173
5.14 The Math Functions 177
5.15 Function Abstraction and Stepwise Refinement 177
5.16 (Optional) Inline Functions 185
Chapter 6 Arrays 199
6.1 Introduction 200
6.2 Array Basics 200
6.3 Passing Arrays to Functions 207
6.4 Returning Arrays from Functions 210
6.5 Searching Arrays 212
6.6 Sorting Arrays 215
6.7 Two-Dimensional Arrays 218
6.8 (Optional) Multidimensional Arrays 225
Chapter 7 Pointers and C-Strings 237
7.1 Introduction 238
7.2 Pointer Basics 238
7.3 Passing Arguments by References with Pointers 241
7.4 Arrays and Pointers 242
7.5 Using const with Pointers 244
7.6 Returning Pointers from Functions 245
7.7 Dynamic Memory Allocation 247
7.8 Case Studies: Counting the Occurrences of Each Letter 249
7.9 Characters and Strings 252
7.10 Case Studies: Checking Palindromes 260
Chapter 8 Recursion 269
8.1 Introduction 270
8.2 Example: Factorials 270
8.3 Example: Fibonacci Numbers 272
8.4 Problem Solving Using Recursion 274
8.5 Recursive Helper Functions 276
8.6 Towers of Hanoi 279
8.7 Recursion versus Iteration 282
PART 2 OBJECT-ORIENTED PROGRAMMING 289
Chapter 9 Objects and Classes 291
9.1 Introduction 292
9.2 Defining Classes for Objects 292
9.3 Constructors 294
9.4 Object Names 294
9.5 Separating Declaration from Implementation 298
9.6 Accessing Object Members via Pointers 300
9.7 Creating Dynamic Objects on Heap 301
9.8 The C++ string Class 301
9.9 Data Field Encapsulation 305
9.10 The Scope of Variables 308
9.11 The this Pointer 310
9.12 Passing Objects to Functions 311
9.13 Array of Objects 313
9.14 Class Abstraction and Encapsulation 315
9.15 Case Study: The Loan Class 315
9.16 Constructor Initializer Lists 319
Chapter 10 More on Objects and Classes 329
10.1 Introduction 330
10.2 Immutable Objects and Classes 330
10.3 Preventing Multiple Declarations 332
10.4 Instance and Static Members 334
10.5 Destructors 337
10.6 Copy Constructors 339
10.7 Customizing Copy Constructors 342
10.8 fri end Functions and fri end Classes 344
10.9 Object Composition 346
10.10 Case Study: The Course Class 347
10.11 Case Study: The StackOfIntegers Class 350
10.12 The C++ vector Class 353
Chapter 11 Inheritance and Polymorphism 361
11.1 Introduction 362
11.2 Base Classes and Derived Classes 362
11.3 Generic Programming 368
11.4 Constructors and Destructors 368
11.5 Redefining Functions 371
11.6 Polymorphism and Virtual Functions 372
11.7 The protected Keyword 375
11.8 Abstract Classes and Pure Virtual Functions 376
11.9 Dynamic Casting 380
Chapter 12 File Input and Output 391
12.1 Introduction 392
12.2 Text I/O 392
12.3 Formatting Output 396
12.4 Member Functions: getline, get, and put 397
12.5 f stream and File Open Modes 400
12.6 Testing Stream States 401
12.7 Binary I/O 403
12.8 Random Access File 410
12.9 Updating Files 413
Chapter 13 Operator Overloading 417
13.1 Introduction 418
13.2 The Rational Class 418
13.3 Operator Functions 423
13.4 Overloading the Shorthand Operators 425
13.5 Overloading the [] Operators 425
13.6 Overloading the Unary Operators 427
13.7 Overloading the ++ and —— Operators 427
13.8 Overloading the << and >> Operators 428
13.9 Object Conversion 430
13.10 The New Rational Class 431
13.11 Overloading the = Operators 438
Chapter 14 Exception Handling 443
14.1 Introduction 444
14.2 Exception-Handling Overview 444
14.3 Exception-Handling Advantages 446
14.4 Exception Classes 447
14.5 Custom Exception Classes 450
14.6 Multiple Catches 455
14.7 Exception Propagation 458
14.8 Rethrowing Exceptions 460
14.9 Exception Specification 461
14.10 When to Use Exceptions 462
PART 3 DATA STRUCTURES 467
Chapter 15 Templates 469
15.1 Introduction 470
15.2 Templates Basics 470
15.3 Example: A Generic Sort 472
15.4 Class Templates 474
15.5 Improving the Stack Class 480
Chapter 16 Linked Lists, Stacks, and Queues 487
16.1 Introduction 488
16.2 Nodes 488
16.3 The Li nkedLi st Class 489
16.4 Implementing Li nkedLi st 492
16.5 Variations of Linked Lists 502
16.6 Implementing Stack Using a Li nkedLi st 502
16.7 Queues 504
16.8 (Optional) Iterators 506
Chapter 17 Trees, Heaps, and Priority Queues 515
17.1 Introduction 516
17.2 Binary Trees 516
17.3 Heaps 524
17.4 Priority Queues 529
Chapter 18 Algorithm Efficiency and Sorting 535
18.1 Introduction 536
18.2 Estimating Algorithm Efficiency 536
18.3 Bubble Sort 541
18.4 Merge Sort 543
18.5 Quick Sort 547
18.6 Heap Sort 551
18.7 External Sort 552
精品推荐
- Northanger Abbey(1818)
- Emma(1815)
- Sense And Sensibility(1811)
- Mansfield Park(1814)
- HUMANITIES THE EVOLUTION OF VALUES
- Pride And Drejudice(1812)
- English
- 企鹅经济学词典 经济学
- 大人的友情 河合隼雄谈友谊
- Computing Concepts
- Advanced Compilpr Design and lmplementation
- 中国商事法律要览
- Introduction to polymers
- CONFICT OF LAWS IN THE WESTERN SOCIALIST AND DEVELOPING COUNTRIES
- Measurement and Research Methods in International Marketing