图书介绍
THINKING IN JAVA 2ND EDITIONpdf电子书版本下载
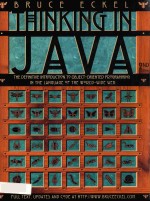
- BRUCE ECKEL 著
- 出版社: PRENTICE HALL
- ISBN:0130273635
- 出版时间:2000
- 标注页数:1130页
- 文件大小:154MB
- 文件页数:1157页
- 主题词:
PDF下载
下载说明
THINKING IN JAVA 2ND EDITIONPDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如 BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
Preface 1
Preface to the 2nd edition 4
Java 2 6
The CD ROM 7
Introduction 9
Prerequisites 9
Learning Java 10
Goals 11
Online documentation 12
Chapters 13
Exercises 19
Multimedia CD ROM 19
Source code 20
Coding standards 22
Java versions 22
Seminars and mentoring 23
Errors 23
Note on the cover design 24
Acknowledgements 25
Internet contributors 28
1:Introduction to Objects 29
The progress of abstraction 30
An object has an interface 32
The hidden implementation 35
Reusing the implementation 37
Inheritance:reusing the interface 38
Is-a vs.is-like-a relationships 42
Interchangeable objects with polymorphism 44
Abstract base classes and interfaces 48
Object landscapes and lifetimes 49
Collections and iterators 51
The singly rooted hierarchy 53
Collection libraries and support for easy collection use 54
The housekeeping dilemma:who should clean up? 55
Exception handling:dealing with errors 57
Multithreading 58
Persistence 60
Java and the Internet 60
What is the Web? 60
Client-side programming 63
Server-side programming 70
A separate arena:applications 71
Analysis and design 71
Phase 0:Make a plan 74
Phase 1:What are we making? 75
Phase 2:How will we build it? 79
Phase 3:Build the core 83
Phase 4:Iterate the use cases 84
Phase 5:Evolution 85
Plans pay off 87
Extreme programming 88
Write tests first 88
Pair programming 90
Why Java succeeds 91
Systems are easier to 91
express and understand 91
Maximal leverage with libraries 92
Error handling 92
Programming in the large 92
Strategies for transition 93
Guidelines 93
Management obstacles 95
Java vs.C++? 97
Summary 98
2:Everything is an Object 101
You manipulate objects with references 101
You must create all the objects 103
Where storage lives 103
Special case:primitive types 105
Arrays in Java 107
You never need to destroy an object 107
Scoping 108
Scope of objects 109
Creating new data types:class 110
Fields and methods 110
Methods,arguments,and return values 112
The argument list 114
Building a Java program 115
Name visibility 115
Using other components 116
The static keyword 117
Your first Java program 119
Compiling and running 121
Comments and embedded documentation 122
Comment documentation 123
Syntax 124
Embedded HTML 125
@see:referring to other classes 125
Class documentation tags 126
Variable documentation tags 127
Method documentation tags 127
Documentation example 128
Coding style 129
Summary 130
Exercises 130
3:Controlling Program Flow 133
Using Java operators 133
Precedence 134
Assignment 134
Mathematical operators 137
Auto increment and decrement 139
Relational operators 141
Logical operators 143
Bitwise operators 146
Shift operators 147
Ternary if-else operator 151
The comma operator 152
String operator + 153
Common pitfalls when using operators 153
Casting operators 154
Java has no “sizeof” 158
Precedence revisited 158
A compendium of operators 159
Execution control 170
true and false 170
if-else 171
Iteration 172
do-while 173
for 173
break and continue 175
switch 183
Summary 187
Exercises 188
4:Initialization & Cleanup 191
Guaranteed initialization with the constructor 191
Method overloading 194
Distinguishing overloaded methods 196
Overloading with primitives 197
Overloading on return values 202
Default constructors 202
The this keyword 203
Cleanup:finalization and garbage collection 207
What is finalize( ) for? 208
You must perform cleanup 209
The death condition 214
How a garbage collector works 215
Member initialization 219
Specifying initialization 221
Constructor initialization 223
Array initialization 231
Multidimensional arrays 236
Summary 239
Exercises 240
5:Hiding the Implementation 243
package:the library unit 244
Creating unique package names 247
A custom tool library 251
Using imports to change behavior 252
Package caveat 254
Java access specifiers 255
“Friendly” 255
public:interface access 256
private:you can’t touch that! 258
protected:“sort of friendly” 260
Interface and implementation 261
Class access 263
Summary 267
Exercises 268
6:Reusing Classes 271
Composition syntax 271
Inheritance syntax 275
Initializing the base class 278
Combining composition and inheritance 281
Guaranteeing proper cleanup 283
Name hiding 286
Choosing composition vs.inheritance 288
protected 290
Incremental development 291
Upcasting 291
Why “upcasting”? 293
The final keyword 294
Final data 294
Final methods 299
Final classes 301
Final caution 302
Initialization and class loading 304
Initialization with inheritance 304
Summary 306
Exercises 307
7:Polymorphism 311
Upcasting revisited 311
Forgetting the object type 313
The twist 315
Method-call binding 315
Producing the right behavior 316
Extensibility 320
Overriding vs.overloading 324
Abstract classes and methods 325
Constructors and polymorphism 330
Order of constructor calls 330
Inheritance and finalize( ) 333
Behavior of polymorphic methods inside constructors 337
Designing with inheritance 339
Pure inheritance vs.extension 341
Downcasting and run-time type identification 343
Summary 346
Exercises 346
8:Interfaces &Inner Classes 349
Interfaces 349
“Multiple inheritance” in Java 354
Extending an interface with inheritance 358
Grouping constants 359
Initializing fields in interfaces 361
Nesting interfaces 362
Inner classes 365
Inner classes and upcasting 368
Inner classes in methods and scopes 370
Anonymous inner classes 373
The link to the outer class 376
static inner classes 379
Referring to the outer class object 381
Reaching outward from a multiply-nested class 383
Inheriting from inner classes 384
Can inner classes be overridden? 385
Inner class identifiers 387
Why inner classes? 388
Inner classes &control frameworks 394
Summary 402
Exercises 403
9:Holding Your Objects 407
Arrays 407
Arrays are first-class objects 409
Returning an array 413
The Arrays class 415
Filling an array 428
Copying an array 429
Comparing arrays 430
Array element comparisons 431
Sorting an array 435
Searching a sorted array 437
Array summary 439
Introduction to containers 439
Printing containers 441
Filling containers 442
Container disadvantage:unknown type 450
Sometimes it works anyway 452
Making a type-conscious ArrayList 454
Iterators 456
Container taxonomy 460
Collection functionality 463
List functionality 467
Making a stack om a LinkedList 471
Making a queue from a LinkedList 472
Set functionality 473
SortedSet 476
Map functionality 476
SortedMap 482
Hashing and hash codes 482
Overriding hashCode() 492
Holding references 495
The WeakHashMap 498
Iterators revisited 500
Choosing an implementation 501
Choosing between Lists 502
Choosing between Sets 506
Choosing between Maps 508
Sorting and searching Lists 511
Utilities 512
Making a Collection or Map unmodiable 513
Synchronizing a Collection or Map 514
Unsupported operations 516
Java 1.0/1.1 containers 519
Vector & Enumeration 519
Hashtable 521
Stack 521
BitSet 522
Summary 524
Exercises 525
10:Error Handling with Exceptions 531
Basic exceptions 532
Exception arguments 533
Catching an exception 534
The try block 535
Exception handlers 535
Creating your own exceptions 537
The exception specication 542
Catching any exception 543
Rethrowing an exception 545
Standard Java exceptions 549
The special case of RuntimeException 550
Performing cleanup with finally 552
What’s finally for? 554
Pitfall:the lost exception 557
Exception restrictions 558
Constructors 562
Exception matching 566
Exception guidelines 568
Summary 568
Exercises 569
11:The Java I/O System 573
The File class 574
A directory lister 574
Checking for and creating directories 578
Input and output 581
Types of InputStream 581
Types of OutputStream 583
Adding attributes and useful interfaces 585
Reading from an InputStream with FilterInputStream 586
Writing to an OutputStream with FilterOutputStream 587
Readers & Writers 589
Sources and sinks of data 590
Modifying stream behavior 591
Unchanged Classes 592
Off by itself:RandomAccessFile 593
Typical uses of I/O streams 594
Input streams 597
Output streams 599
A bug? 601
Piped streams 602
Standard I/O 602
Reading from standard input 603
Changing System.out to a PrintWriter 604
Redirecting standard I/O 604
Compression 606
Simple compression with GZIP 607
Multifile storage with Zip 608
Java ARchives (JARs) 611
Object serialization 613
Finding the class 618
Controlling serialization 619
Using persistence 630
Tokenizing input 639
StreamTokenizer 639
StringTokenizer 642
Checking capitalization style 645
Summary 655
Exercises 656
12:Run-time Type Identification 659
The need for RTTI 659
The Class object 662
Checking before a cast 665
RTTI syntax 674
Reflection:run-time class information 677
A class method extractor 679
Summary 685
Exercises 686
13 Creating Windows & Applets 689
The basic applet 692
Applet restrictions 692
Applet advantages 693
Application frameworks 694
Running applets inside a Web browser 695
Using Appletviewer 698
Testing applets 698
Running applets from the command line 700
A display framework 702
Using the Windows Explorer 705
Making a button 706
Capturing an event 707
Text areas 711
Controlling layout 712
BorderLayout 713
FlowLayout 714
GridLayout 715
GridBagLayout 716
Absolute positioning 716
BoxLayout 717
The best approach? 721
The Swing event model 722
Event and listener types 723
Tracking multiple events 730
A catalog of Swing components 734
Buttons 734
Icons 738
Tool tips 740
Text fields 740
Borders 743
JScrollPanes 744
A mini-editor 747
Check boxes 748
Radio buttons 750
Combo boxes(drop-down lists) 751
List boxes 753
Tabbed panes 755
Message boxes 756
Menus 759
Pop-up menus 766
Drawing 768
Dialog Boxes 771
File dialogs 776
HTML on Swing components 779
Sliders and progress bars 780
Trees 781
Tables 784
Selecting Look & Feel 787
The clipboard 790
Packaging an applet into a JAR file 793
Programming techniques 794
Binding events dynamically 794
Separating business logic from UI logic 796
A canonical form 799
Visual programming and Beans 800
What is a Bean? 801
Extracting BeanInfo with the Introspector 804
A more sophisticated Bean 811
Packaging a Bean 816
More complex Bean support 818
More to Beans 819
Summary 819
Exercises 820
14:Multiple Threads 825
Responsive user interfaces 826
Inheriting from Thread 828
Threading for a responsive interface 831
Combining the thread with the main class 834
Making many threads 836
Daemon threads 840
Sharing limited resources 842
Improperly accessing resources 842
How Java shares resources 848
JavaBeans revisited 854
Blocking 859
Becoming blocked 860
Deadlock 872
Priorities 877
Reading and setting priorities 878
Thread groups 882
Runnable revisited 891
Too many threads 894
Summary 899
Exercises 901
15:Distributed Computing 903
Network programming 904
Identifying a machine 905
Sockets 909
Serving multiple clients 917
Datagrams 923
Using URLs from within an applet 923
More to networking 926
Java Database Connectivity (JDBC) 927
Getting the example to work 931
A GUI version of the lookup program 935
Why the JDBC API seems so complex 938
A more sophisticated example 939
Servlets 948
The basic servlet 949
Servlets and multithreading 954
Handling sessions with servlets 955
Running the servlet examples 960
Java Server Pages 960
Implicit objects 962
JSP directives 963
JSP scripting elements 964
Extracting fields and values 966
JSP page attributes and scope 968
Manipulating sessions in JSP 969
Creating and modifying cookies 971
JSP summary 972
RMI (Remote Method Invocation) 973
Remote interfaces 973
Implementing the remote interface 974
Creating stubs and skeletons 978
Using the remote object 979
CORBA 980
CORBA fundamentals 981
An example 983
Java Applets and CORBA 989
CORBA vs.RMI 989
Enterprise JavaBeans 990
JavaBeans vs.EJBs 991
The EJB specification 992
EJB components 993
The pieces of an EJB component 994
EJB operation 995
Types of EJBs 996
Developing an EJB 997
EJB summary 1003
Jini:distributed services 1003
Jini in context 1003
What is Jini? 1004
How Jini works 1005
The discovery process 1006
The join process 1006
The lookup process 1007
Separation of interface and implementation 1008
Abstracting distributed systems 1009
Summary 1010
Exercises 1010
A:Passing &Returning Objects 1013
Passing references around 1014
Aliasing 1014
Making local copies 1017
Pass by value 1018
Cloning objects 1018
Adding cloneability to a class 1020
Successful cloning 1022
The effect of Object.clone() 1025
Cloning a composed object 1027
A deep copy with ArrayList 1030
Deep copy via serialization 1032
Adding cloneabilityfurther down a hierarchy 1034
Why this strange design? 1035
Controlling cloneability 1036
The copy constructor 1042
Read-only classes 1047
Creating read-only classes 1049
The drawback to immutability 1050
Immutable Strings 1052
The String and StringBuffer classes 1056
Strings are special 1060
Summary 1060
Exercises 1062
B :The Java Native Interface (JNI) 1065
Calling a native method 1066
The header file generator:javah 1067
Name mangling and function signatures 1068
Implementing your DLL 1068
Accessing JNI functions:the JNIEnv argument 1069
Accessing Java Strings 1071
Passing and using Java objects 1071
JNI and Java exceptions 1074
JNI and threading 1075
Using a preexisting code base 1075
Additional information 1076
C:Java Programming Guidelines 1077
Design 1077
Implementation 1084
D:Resources 1091
Software 1091
Books 1091
Analysis & design 1093
Python 1095
My own list of books 1096
Index 1099
精品推荐
- Northanger Abbey(1818)
- Emma(1815)
- Sense And Sensibility(1811)
- Mansfield Park(1814)
- HUMANITIES THE EVOLUTION OF VALUES
- Pride And Drejudice(1812)
- English
- 企鹅经济学词典 经济学
- 大人的友情 河合隼雄谈友谊
- Computing Concepts
- Advanced Compilpr Design and lmplementation
- 中国商事法律要览
- Introduction to polymers
- CONFICT OF LAWS IN THE WESTERN SOCIALIST AND DEVELOPING COUNTRIES
- Measurement and Research Methods in International Marketing